1.简介
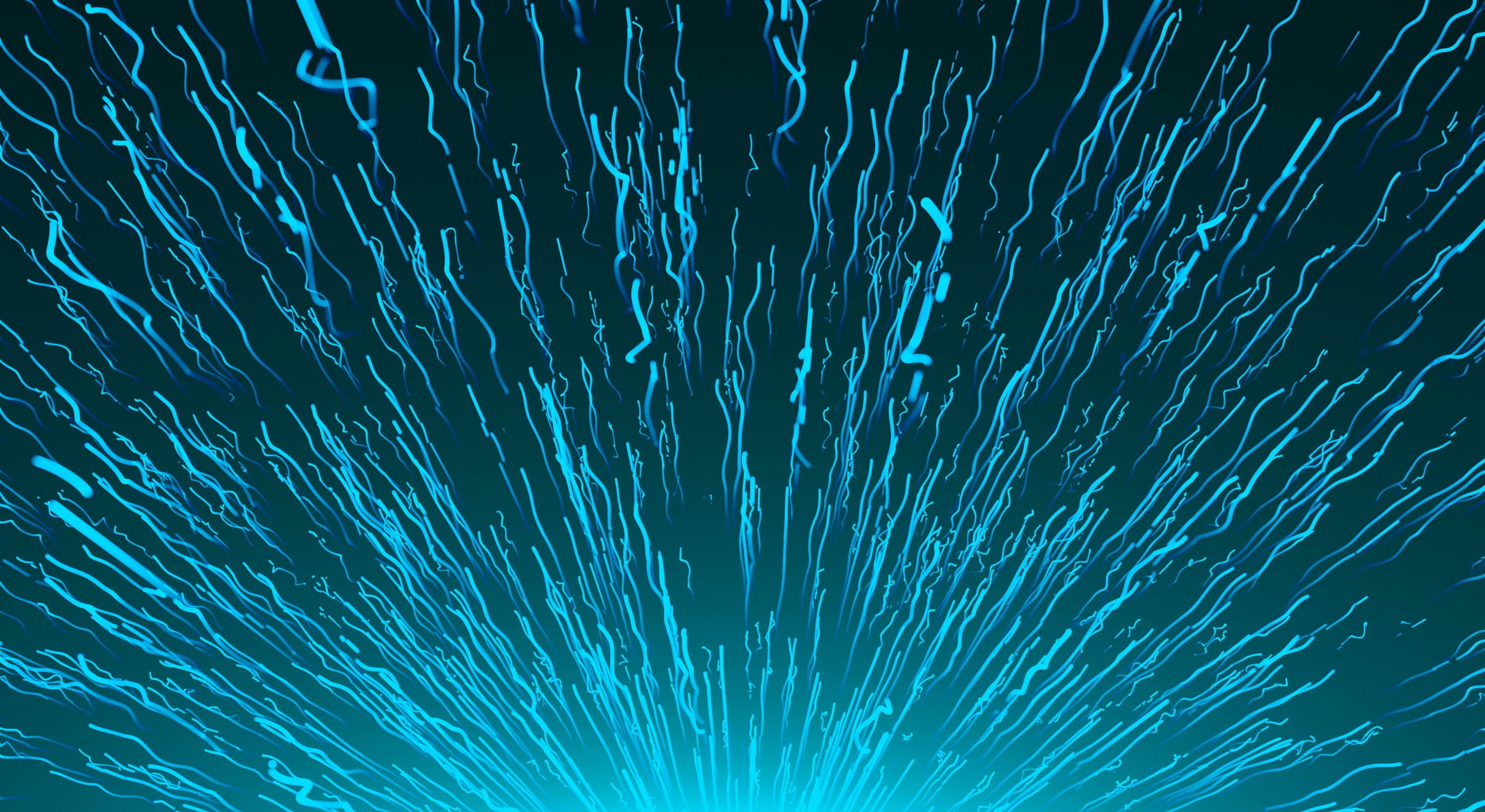
在本篇文章中,我们将介绍Spring Boot及其注解驱动的开发模式。Spring Boot是一个非常受欢迎的Java框架,它可以帮助开发者更快速、更轻松地构建基于Spring的应用程序。Spring Boot通过自动配置、约定优于配置的理念以及各种starter依赖,大大简化了Spring应用程序的开发过程。
Spring Boot采用了注解驱动的开发模式,这种模式允许开发者通过在代码中添加注解来实现功能,而无需编写大量的样板代码。注解可以帮助我们实现依赖注入、自动配置和其他高级功能。这种简洁的方式使得开发者能够专注于编写核心业务逻辑,而不是花费大量时间在配置和管理上。
本文将分析不同类型的注解,包括配置类注解、组件扫描注解、依赖注入注解等,并通过实例来演示它们的用法。
2.核心注解
2.1 @SpringBootApplication
@SpringBootApplication注解是Spring Boot应用程序的核心注解,通常位于主类上。它包含了@Configuration、@EnableAutoConfiguration和@ComponentScan三个注解,因此具有这三个注解的功能。
@SpringBootApplication
public class Application {
public static void main(String[] args) {
SpringApplication.run(Application.class, args);
}
}
2.2 @Configuration
@Configuration注解用于表示一个类是配置类,这意味着该类可以包含定义@Bean的方法。这些@Bean方法将由Spring容器负责实例化、配置和管理对象。
@Configuration
public class AppConfig {
@Bean
public MyService myService() {
return new MyServiceImpl();
}
}
2.3 @EnableAutoConfiguration
@EnableAutoConfiguration注解告诉Spring Boot根据添加的依赖自动配置项目。例如,如果项目中包含了spring-boot-starter-web依赖,Spring Boot将自动配置一个嵌入式的Tomcat服务器和其他与Web开发相关的配置。
@EnableAutoConfiguration
public class Application {
public static void main(String[] args) {
SpringApplication.run(Application.class, args);
}
}
2.4 @ComponentScan
@ComponentScan注解告诉Spring从指定的包开始扫描组件(如:@Component, @Service, @Controller等)。如果未指定包路径,将从声明@ComponentScan的类所在的包开始扫描。
@ComponentScan(basePackages = "com.example.demo")
public class Application {
public static void main(String[] args) {
SpringApplication.run(Application.class, args);
}
}
2.5 @Bean
@Bean注解用于声明一个方法返回的对象应由Spring容器管理。这意味着Spring将负责创建、配置和销毁这些对象。@Bean通常与@Configuration一起使用,但也可以在其他类型的组件中使用。
@Configuration
public class AppConfig {
@Bean
public MyService myService() {
return new MyServiceImpl();
}
}
3.控制器与请求映射注解
3.1 @Controller
@Controller注解用于声明一个类是Spring MVC的控制器。@Controller类通常与@RequestMapping注解一起使用,用于定义处理HTTP请求的方法。
@Controller
public class MyController {
@RequestMapping("/hello")
public String hello() {
return "hello";
}
}
3.2 @RestController
@RestController注解是@Controller和@ResponseBody注解的组合,用于声明一个类是RESTful风格的控制器。它将自动将方法的返回值转换为JSON或XML等格式,并将其写入HTTP响应中。
@RestController
public class MyRestController {
@RequestMapping("/hello")
public String hello() {
return "hello";
}
}
3.3 @RequestMapping
@RequestMapping注解用于定义处理特定HTTP请求的方法。可以指定请求的URL、HTTP方法和请求头等属性。
@Controller
public class MyController {
@RequestMapping(value = "/hello", method = RequestMethod.GET)
public String hello() {
return "hello";
}
}
3.4 @GetMapping,@PostMapping,@PutMapping, @DeleteMapping, @PatchMapping
这些注解是@RequestMapping的快捷方式,分别对应GET、POST、PUT、DELETE和PATCH HTTP方法。
@RestController
public class MyRestController {
@GetMapping("/hello")
public String hello() {
return "hello";
}
@PostMapping("/submit")
public String submit(@RequestBody MyData data) {
// 处理提交的数据
return "success";
}
}
3.5@PathVariable,@RequestParam,@RequestHeader, @RequestBody, @RequestAttribute
- @PathVariable:用于将URL路径中的变量绑定到方法参数。
- @RequestParam:用于将HTTP请求参数绑定到方法参数。
- @RequestHeader:用于将HTTP请求头信息绑定到方法参数。
- @RequestBody:用于将HTTP请求体的内容绑定到方法参数。通常与JSON、XML等数据格式结合使用。
- @RequestAttribute:用于将请求作用域中的属性绑定到方法参数。
@RestController
public class MyRestController {
@GetMapping("/users/{userId}")
public User getUser(@PathVariable("userId") Long userId) {
// 查询并返回用户信息
}
@GetMapping("/search")
public List<User> searchUsers(@RequestParam("keyword") String keyword) {
// 根据关键字搜索并返回用户列表
}
@PostMapping("/submit")
public String submit(@RequestHeader("Content-Type") String contentType, @RequestBody MyData data) {
// 处理提交的数据
return "success";
}
}
4.服务与数据访问注解
4.1 @Service
@Service注解用于表示一个类是业务逻辑层的组件。它通常与@Repository或@Autowired等注解一起使用,用于处理核心业务功能和与数据访问层的交互。
@Service
public class UserService {
@Autowired
private UserRepository userRepository;
public User findUserById(Long id) {
return userRepository.findById(id);
}
}
4.2 @Repository
@Repository注解用于声明一个类是数据访问层组件。通常用于DAO(Data Access Object)类,并与数据库相关的操作一起使用。
@Repository
public class UserRepository {
// 数据库访问操作
}
4.3 @Autowired
@Autowired注解用于自动装配Spring容器中的Bean。它可以用于字段、构造函数和方法参数,以便将其他Bean注入到当前Bean。
@Service
public class UserService {
@Autowired
private UserRepository userRepository;
// ...
}
4.4 @Qualifier
@Qualifier注解用于在多个具有相同类型的Bean中,指定需要注入的Bean。它与@Autowired注解一起使用。
@Service
public class UserService {
@Autowired
@Qualifier("userRepository")
private UserRepository userRepository;
// ...
}
4.5 @Resource
@Resource注解用于注入Spring容器中的Bean。它是Java EE的标准注解,与@Autowired功能类似,但是@Resource默认按名称注入,而@Autowired默认按类型注入。
@Service
public class UserService {
@Resource(name = "userRepository")
private UserRepository userRepository;
// ...
}
4.6 @Transactional
@Transactional注解用于声明一个方法或类需要数据库事务支持。它可以确保在方法执行过程中,如果出现异常,会回滚数据库操作,否则会提交事务。
@Service
public class UserService {
@Autowired
private UserRepository userRepository;
@Transactional
public void updateUser(User user) {
// 更新用户信息
userRepository.update(user);
// 其他数据库操作
}
}
5.配置属性注解
5.1 @ConfigurationProperties
@ConfigurationProperties注解用于将配置文件(如application.properties或application.yml)中的属性绑定到一个Java类上。这种方式使得使用配置属性更加简洁和方便。
首先,需要在pom.xml中添加spring-boot-configuration-processor依赖:
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-configuration-processor</artifactId>
</dependency>
然后,创建一个类,并使用@ConfigurationProperties注解:
@ConfigurationProperties(prefix = "app")
public class AppConfig {
private String name;
private String version;
// Getter and setter methods
}
在配置文件中,添加相应的属性:
app.name=MyApplication
app.version=1.0.0
5.2 @PropertySource
@PropertySource注解用于将一个外部的配置文件加载到Spring环境中。这样,可以在@Configuration类中使用这些属性。
@Configuration
@PropertySource("classpath:custom.properties")
public class CustomConfig {
// ...
}
在这个例子中,custom.properties文件位于项目的classpath下。
5.3 @Value
@Value注解用于将配置文件中的单个属性值注入到Bean中。它可以用于字段、构造函数参数和方法参数。
@Service
public class MyService {
@Value("${app.name}")
private String appName;
@Value("${app.version}")
private String appVersion;
public void printAppInfo() {
System.out.println("App Name: " + appName);
System.out.println("App Version: " + appVersion);
}
}
6.条件注解
6.1 @Conditional
@Conditional注解用于在满足某个特定条件时才创建并注册Bean。需要创建一个实现Condition接口的类,并重写matches方法,根据条件返回true或false。
public class CustomCondition implements Condition {
@Override
public boolean matches(ConditionContext context, AnnotatedTypeMetadata metadata) {
// Check condition
return true; // or false
}
}
在配置类或@Bean方法上使用@Conditional注解:
@Configuration
@Conditional(CustomCondition.class)
public class CustomConfig {
// ...
}
6.2 @ConditionalOnProperty
@ConditionalOnProperty注解用于当指定的配置属性满足某个条件时,才创建并注册Bean。
@Bean
@ConditionalOnProperty(name = "app.enable-feature", havingValue = "true")
public FeatureService featureService() {
return new FeatureService();
}
6.3 @ConditionalOnClass
@ConditionalOnClass注解用于当指定的类在类路径上存在时,才创建并注册Bean。
@Bean
@ConditionalOnClass(name = "com.example.SomeClass")
public SomeService someService() {
return new SomeService();
}
6.4 @ConditionalOnMissingClass
@ConditionalOnMissingClass注解用于当指定的类在类路径上不存在时,才创建并注册Bean。
@Bean
@ConditionalOnMissingClass("com.example.SomeClass")
public AnotherService anotherService() {
return new AnotherService();
}
6.5 @ConditionalOnBean
@ConditionalOnBean注解用于当指定类型的Bean存在时,才创建并注册Bean。
@Bean
@ConditionalOnBean(DataSource.class)
public JdbcTemplate jdbcTemplate(DataSource dataSource) {
return new JdbcTemplate(dataSource);
}
6.6 @ConditionalOnMissingBean
@ConditionalOnMissingBean注解用于当指定类型的Bean不存在时,才创建并注册Bean。
@Bean
@ConditionalOnMissingBean(CacheManager.class)
public CacheManager defaultCacheManager() {
return new SimpleCacheManager();
}
7.缓存注解
7.1 @Cacheable
@Cacheable 注解用于在方法上指定缓存策略。当调用该方法时,会先查找缓存,如果缓存中有数据,则直接返回缓存中的数据;如果缓存中没有数据,则调用方法并将方法返回的结果放入缓存。
@Service
public class ProductService {
@Cacheable(value = "products", key = "#id")
public Product getProduct(Long id) {
// Fetch product from the database
return product;
}
}
7.2 @CachePut
@CachePut 注解用于在方法上指定更新缓存的策略。当调用该方法时,会先执行方法,然后将方法返回的结果更新到缓存中。
@Service
public class ProductService {
@CachePut(value = "products", key = "#product.id")
public Product updateProduct(Product product) {
// Update product in the database
return updatedProduct;
}
}
7.3 @CacheEvict
@CacheEvict 注解用于在方法上指定清除缓存的策略。当调用该方法时,会删除与指定key相关的缓存。
@Service
public class ProductService {
@CacheEvict(value = "products", key = "#id")
public void deleteProduct(Long id) {
// Delete product from the database
}
}
7.4 @Caching
@Caching 注解用于组合多个缓存注解。这在需要对一个方法应用多个缓存操作时非常有用。
@Service
public class ProductService {
@Caching(
put = @CachePut(value = "products", key = "#product.id"),
evict = @CacheEvict(value = "products", key = "#product.oldId")
)
public Product changeProductId(Product product) {
// Change product id in the database
return updatedProduct;
}
}
7.5 @EnableCaching
@EnableCaching 注解用于启用Spring缓存支持。通常在主配置类上添加此注解。
@Configuration
@EnableCaching
public class AppConfig {
// ...
}
8.异步与定时任务注解
8.1 @Async
@Async 注解用于在方法上指定该方法应异步执行。这意味着当调用该方法时,它将在一个新的线程上运行,而不是在调用者的线程上执行。
@Service
public class EmailService {
@Async
public void sendEmail(String recipient, String message) {
// Send email asynchronously
}
}
8.2 @EnableAsync
@EnableAsync 注解用于启用异步方法执行支持。通常在主配置类上添加此注解。
@Configuration
@EnableAsync
public class AppConfig {
// ...
}
8.3 @Scheduled
@Scheduled 注解用于在方法上指定该方法应定期执行。可以通过提供固定延迟、固定频率或基于Cron表达式的计划来设置任务执行计划。
@Service
public class ScheduledTaskService {
@Scheduled(fixedRate = 60000)
public void performTask() {
// Task to be performed every 60,000 milliseconds (1 minute)
}
@Scheduled(cron = "0 0 12 * * ?")
public void performTaskAtNoon() {
// Task to be performed every day at noon
}
}
8.4 @EnableScheduling
@EnableScheduling 注解用于启用定时任务支持。通常在主配置类上添加此注解。
@Configuration
@EnableScheduling
public class AppConfig {
// ...
}
9.测试相关注解
9.1 @SpringBootTest
@SpringBootTest 注解用于表示这是一个 Spring Boot 集成测试。它将启动一个完整的 Spring ApplicationContext,并可自动配置已添加的组件。这使得测试能够使用 Spring Boot 功能来运行集成测试。
@RunWith(SpringRunner.class)
@SpringBootTest
public class MyApplicationTests {
@Test
public void contextLoads() {
// Test if the application context is loaded correctly
}
}
9.2 @RunWith
@RunWith 注解用于在 JUnit 中指定测试运行器。在 Spring Boot 集成测试中,通常使用 SpringRunner.class 作为运行器。
@RunWith(SpringRunner.class)
@SpringBootTest
public class MyApplicationTests {
// ...
}
9.3 @MockBean
@MockBean 注解用于创建一个模拟的 Bean,以替换实际的 Bean。这对于在测试环境中模拟外部服务或资源非常有用。
@RunWith(SpringRunner.class)
@SpringBootTest
public class MyServiceTests {
@Autowired
private MyService myService;
@MockBean
private ExternalService externalService;
@Test
public void testMyService() {
// Configure mock behavior for externalService and test myService
}
}
9.4 @TestPropertySource
@TestPropertySource 注解用于指定测试期间使用的属性文件。这允许使用特定于测试的配置。
@RunWith(SpringRunner.class)
@SpringBootTest
@TestPropertySource(locations = "classpath:test.properties")
public class MyApplicationTests {
// ...
}
9.5 @ActiveProfiles
@ActiveProfiles 注解用于指定在测试期间激活的配置文件。这允许在不同环境(如开发、测试和生产)之间切换配置。
javaCopy code@RunWith(SpringRunner.class)
@SpringBootTest
@ActiveProfiles("test")
public class MyApplicationTests {
// ...
}
10.总结
10.1 Spring Boot注解的优势
Spring Boot 注解具有以下优势:
- 简化开发:Spring Boot 注解简化了开发流程,让开发人员可以专注于业务逻辑而无需过多关注底层配置。
- 代码清晰:注解使得代码更清晰,易于理解和维护,因为它们表明了代码的目的和功能。
- 高度可定制:Spring Boot 提供了大量的注解,使得开发者可以根据需要定制应用程序的行为和配置。
- 易于集成:Spring Boot 注解使得与其他库和框架的集成变得容易,提高了开发效率。
10.2 注意事项与最佳实践
- 不要过度使用注解:虽然注解可以简化代码和配置,但过度使用可能会导致代码可读性降低。在合适的场景下使用注解,并保持代码简洁。
- 了解注解的作用:在使用注解之前,确保了解它们的具体作用和使用场景。这有助于避免潜在的问题和错误。
- 注解组合:在某些情况下,可以将多个注解组合使用,以提高代码的可读性和可维护性。例如,使用 @RestController 代替 @Controller 和 @ResponseBody 的组合。
- 关注性能:在使用注解时,要注意性能问题。例如,当使用缓存注解时,要确保适当配置缓存策略,以避免资源浪费和性能下降。
10.3 未来发展展望
随着 Spring Boot 持续发展,我们可以期待更多有用的注解被引入,进一步简化开发流程和提高开发效率。此外,随着 Java 和 Spring Boot 社区的发展,对注解的最佳实践和使用方法的研究也将不断深入,为开发者提供更多的指导。